Hello, this tutorial will show you how to create a project from scratch without using a project template. First you need to download the latest version from github github.com/Andy16823/GFX/releases/latest. Once you have downloaded the latest version, we will create a new project in Visual Studio. For this tutorial we will create a Winforms C# project using .Net Framework 4.8, dont worry we will not use a form within this project, we will use the Win32 window from the framework itself.
Once you have created your project, you will need to set up the dependencies. We will start with the files from the release you have downloaded. Extract the zip file and add the following dll to your project
Create the Game Class
After adding the Genesis.dll to the project, you must remove the existing form from the project. Then we will create a new class called "MyGame" and make it inherit from the "Game" class from the genesis.core namespace. Intelisense will now complain that we are missing the constructor. Let intelisense create it for you. Your class should now look like this
Display All
Now it is time to fill the class. But before we start, we need to create a folder inside our project called "Resources". Once this is done we will tell our game to load the assets from this folder and set the target fps to 200
In order to use physics in our game, we need to create a physics handler, but don't worry, it's not as complicated as you might think. It is just one line of code
Then we need to create the scene we want to render. You can think of a scene as a level. Everything in this scene will be rendered and updated within the game loop
As you can see, we also assigned a camera and our previously created physichandler to this scene. This means that each scene is independent of the others. You can create 2D and 3D scenes within the same project. In the next step we will create our first game element, a simple sprite. To do this we need to copy the image for the sprite into the Resources directory we created earlier. Once you have placed your image in this folder, make sure that you tell Visual Studio to copy the image to the target directory within the build. This option can be found within the properties of the file. (see screenshot)
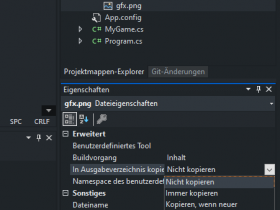
Once you have copied the file into the resource folder, we will add the code to add the sprite to the level.
As you can see with the last parameter inside the sprite constructor, we can get any resource from this folder just by passing the game and the extension. Now we can finally add the created scene to the game and load it. Only 1 scene can be active at a time in the game!
In order to have some control over our game, we should add some events. We do this in gfx using lambda expressions. Let us create a simple callback for the update. We can do this easily like this
So our game class is done for now. In total, your game class should look like this
Display All
Create the Project.cs
Ok, nice, but our game will not run yet. We need to change the code in Progamm.cs first. You can simply replace the Main function within the following code in your program.cs
Display All
Change the Project Plattform
Ok, now that we have our MyGame and our programming class, we could run the game, right? well, not yet. We need to change the build CPU architecture to x64. Within Visual Studio the default architecture is set to "Any CPU" but the engine requires a 64 bit CPU. So we need to change that. To do this, click on "Configuration Manager" in the combo box where "Any CPU" is displayed (see screenshot).
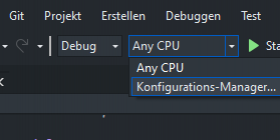
A new window will pop up and we will click on <New> in the Platform drop down menu.
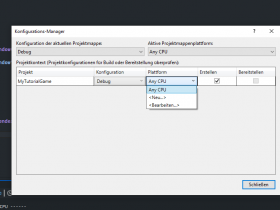
Within the first combobox in the new window we will select x64 and click on ok.
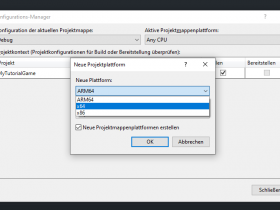
The Native Libarys
Now we need to do one more step before we can run the build. We need to copy the native libraries from the release folder you downloaded into the project, and also have Visual Studio copy them into the output directory. These files need to be in the same folder as the exe of your game. Make sure you copy them to the root directory, not to any folder. The ddl you need to copy are libbulletc-windows-x64.dll and libbulletc.dll.
Press Start and run the game now! In addition to this you also can find the tutorial project here: GFX/Examples/MyTutorialGame at main · Andy16823/GFX (github.com) and can download it here: gfx-engine.org/wp-content/uploads/2024/07/MyTutorialGame.zip
Once you have created your project, you will need to set up the dependencies. We will start with the files from the release you have downloaded. Extract the zip file and add the following dll to your project
Genesis.dll
Create the Game Class
After adding the Genesis.dll to the project, you must remove the existing form from the project. Then we will create a new class called "MyGame" and make it inherit from the "Game" class from the genesis.core namespace. Intelisense will now complain that we are missing the constructor. Let intelisense create it for you. Your class should now look like this
C Source Code
- using Genesis.Core;
- using Genesis.Graphics;
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using System.Threading.Tasks;
- namespace MyTutorialGame
- {
- public class MyGame : Game
- {
- public MyGame(IRenderDevice renderDevice, Viewport viewport) : base(renderDevice, viewport)
- {
- }
- }
- }
Once you have copied the file into the resource folder, we will add the code to add the sprite to the level.
C Source Code
In order to have some control over our game, we should add some events. We do this in gfx using lambda expressions. Let us create a simple callback for the update. We can do this easily like this
So our game class is done for now. In total, your game class should look like this
C Source Code
- using Genesis.Core;
- using Genesis.Core.Behaviors.Physics2D;
- using Genesis.Core.GameElements;
- using Genesis.Graphics;
- using Genesis.Graphics.Physics;
- using Genesis.Math;
- using Genesis.Physics;
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using System.Threading.Tasks;
- namespace MyTutorialGame
- {
- public class MyGame : Game
- {
- public MyGame(IRenderDevice renderDevice, Viewport viewport) : base(renderDevice, viewport)
- {
- // Load the textures and set the target fps
- this.AssetManager.LoadTextures();
- this.TargetFPS = 200;
- // Create an physics handler for the scene
- var physicsHandler = new PhysicsHandler2D(0f, 0f);
- // Setup the Scene with an sun, layer, camera and physics handler
- var scene = new Scene("MyTestScene");
- scene.AddLayer("BaseLayer");
- scene.Camera = new Camera(viewport, -1f, 1f);
- scene.PhysicHandler = physicsHandler;
- // Create your game elements
- var sprite = new Sprite("sprite", new Vec3(0, 0), new Vec3(100, 100), this.AssetManager.GetTexture("gfx.png"));
- var collider = sprite.AddBehavior<BoxCollider>(new BoxCollider(physicsHandler));
- collider.CreateCollider();
- scene.AddGameElement("BaseLayer", sprite);
- // Add the Scene to the game and load it.
- this.AddScene(scene);
- this.LoadScene("MyTestScene");
- this.OnUpdate += (g, r) =>
- {
- Console.WriteLine("Hello World");
- };
- }
- }
- }
Create the Project.cs
Ok, nice, but our game will not run yet. We need to change the code in Progamm.cs first. You can simply replace the Main function within the following code in your program.cs
C Source Code
- static void Main()
- {
- // Create an window Handle
- Genesis.Core.Window window = new Genesis.Core.Window();
- Viewport viewport = new Viewport(1280, 720);
- var handle = window.CreateWindowHandle("Hello Window", viewport);
- // Create Render Settings for the renderer
- var renderSettings = new RenderSettings()
- {
- gamma = 0.5f
- };
- // Create the game
- var game = new MyGame(new BetaRenderer(handle, renderSettings), viewport, window);
- // Show and Run the Game
- window.RunGame(game);
- }
Change the Project Plattform
Ok, now that we have our MyGame and our programming class, we could run the game, right? well, not yet. We need to change the build CPU architecture to x64. Within Visual Studio the default architecture is set to "Any CPU" but the engine requires a 64 bit CPU. So we need to change that. To do this, click on "Configuration Manager" in the combo box where "Any CPU" is displayed (see screenshot).
A new window will pop up and we will click on <New> in the Platform drop down menu.
Within the first combobox in the new window we will select x64 and click on ok.
The Native Libarys
Now we need to do one more step before we can run the build. We need to copy the native libraries from the release folder you downloaded into the project, and also have Visual Studio copy them into the output directory. These files need to be in the same folder as the exe of your game. Make sure you copy them to the root directory, not to any folder. The ddl you need to copy are libbulletc-windows-x64.dll and libbulletc.dll.
Press Start and run the game now! In addition to this you also can find the tutorial project here: GFX/Examples/MyTutorialGame at main · Andy16823/GFX (github.com) and can download it here: gfx-engine.org/wp-content/uploads/2024/07/MyTutorialGame.zip